Automating VM Management: Writing Scripts to Power VMs Up and Down
In this article I write about how I created powershell scripts for starting up and shutting down VM's in VMware. I provide code snippets and explain in a simple way of what is going on.
2/21/20255 min read
Reasoning
I only have a few hours to dedicate to working on my homelab after work. For context, my homelab consists of 7 virtual machines (VMs) in VMware, and because my PC is not a server, it is not running 24/7. Therefore, every day after work, I would have to manually go to every VM and turn them on. After a couple of hours, I would then have to shut every one of them down individually, which was more than one mouse click per VM. All of this was getting pretty monotonous and I was getting tired of it. So I did some research, and I decided to automate this process.
Below is an image of the drop down menu, I had to navigate every time I wanted to shut down the Guest VM:
Action
After doing research, it turns out that VMware has built-in tools that allow you to interact with VMs from the command line on the host OS, which allows for automation. The tool I focused on for the automation script was vmrun.exe. This tool includes power commands (start, stop), snapshot commands (revertToSnapshot), Guest OS commands, and more.
As a per-requisite for vmrun.exe to interact with the guest OS cleanly, VMware tools needs to be installed first on each VM. I had 3 different types of VM operating systems:
pfSense → FreeBSD
Kali Linux → Debian-based Linux
Windows → Windows NT-based OS
This meant that I had to check and install VMware tools in three different ways.
For Windows, you can install VMware tools through the drop down menu in VMware. To verify if VMware tools was installed, I used this command:
Get-WmiObject -Class Win32_Product | Where-Object { $_.Name -like "VMware Tools*" }
For Debian, you can also install through the drop down menu. To verify, I used this command:
vmware-toolbox-cmd -v
For FreeBSD, I could not install VMware tools through VMware. I had to install it with another machine accessing the web interface of Pfsense as shown below:
You can also verify if VMware tools is installed on a VM if you see reinstall VMware tools in the VM drop down menu as shown below:
Automation Scripts
Startup Script
For the startup script, I first have to open VMware using Start-Process and point to where VMware is installed.
I instructed the script to wait for 5 seconds using Start-Sleep 5 to give time for the VMware application to up.
I created a variable that lists the path to the vmrun.exe tool.
I created an array of all the VM paths I wanted to start.
Next, I defined a function that determines which VMs are currently running. It returns True if VMs are already running, and False if no VMs are running.
In line 27, the & (call operator) ensures vmrun list runs as an external command. In vmrun.exe, the list command lists the currently running VMs. So, the variable $runningVMs stores a list of all the currently running VMs.
In line 28, it returns true if the VM that is running matches the VM path, and false if it does not match. Also, regular expressions (regex) use special characters (like . \ * + ? | etc.) to define patterns. If a string contains these characters and you use it directly in a regex match, it may behave unexpectedly. The [regex]::Escape() method is used in PowerShell to safely escape special characters in a string, ensuring that they are treated as literal characters in a regular expression.
Lastly, I created a loop that loops through the VMs I want to start, and compares them to the currently running VMs to determine what action to take next. If the function test-VMRunning returns true, then it outputs "Vm already running" and loops to the next item($vm) in the list. -vmxPath is the parameter and intakes the VMs specified in $vmlist created in line 12. If it returns false, then it starts the VM with the $vmrun tool. Then, I output to the terminal that the script is finished.
Shutdown Script
The shutdown script is much simpler since I do not use regex to determine if a VM is already running or not. Like the previous script, I first store the vmrun.exe tool in a variable.
I create a list of the output of the vmrun.exe list command named $listVMs.
Then, I create another list called $runningVMs which is the output of slicing $listVM to skip the first line of the list, which reads "Total running VMs: X". I do this to create a list of only running VMs, and the first line is not a running VM so it would've caused an error.
[1..($listVMs.Length - 1)] is a way of slicing through the $listVMs array starting from the 1 position to the end of array. You can slice an array using the .. (range operator).
Lastly, I create a for loop that gracefully stops each running VM using the $vmrun stop "$vm" soft command, and indicate that the script has been finished.
It turns out that, like a physical machine, virtual machines also have to be powered off gracefully. If not, then data corruption can occur, since it is the equivalent to pulling the power cord on a physical machine. In vmrun, the command to power off the machine gracefully is vmrun.exe stop pathToVM soft.
Both scripts are available on my Github site. Every environment is different, so the only things you need to change is the path to VMware, vmrun.exe, and paths to VMs in $vmList. Feel free to use them to make your life more convenient.
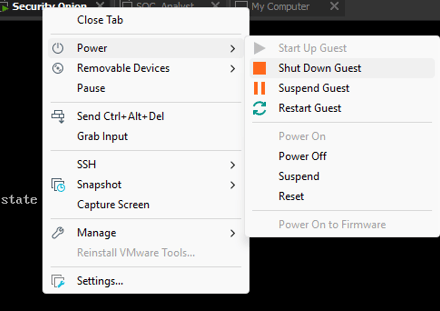
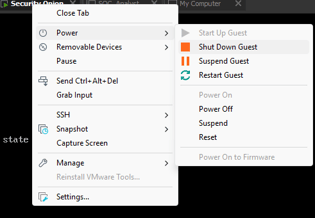
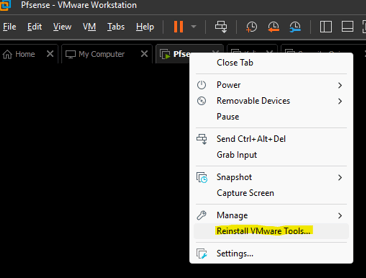
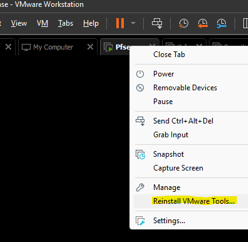
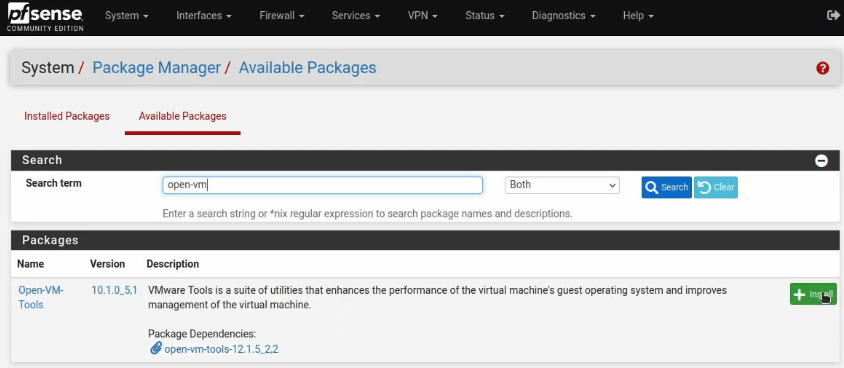
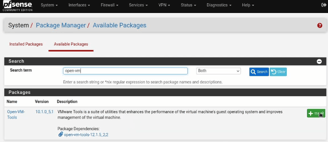
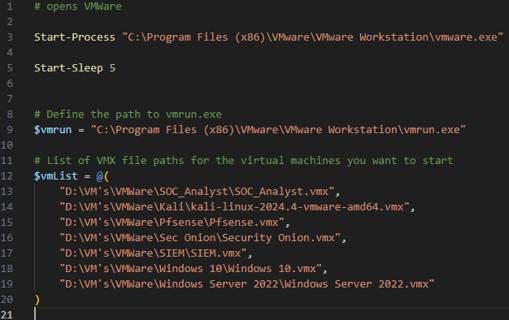
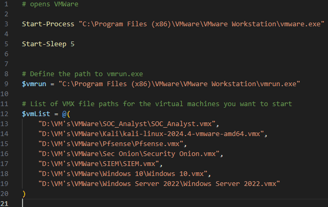
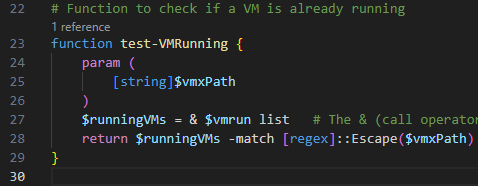
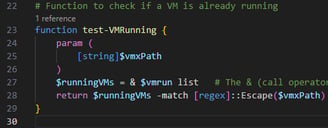
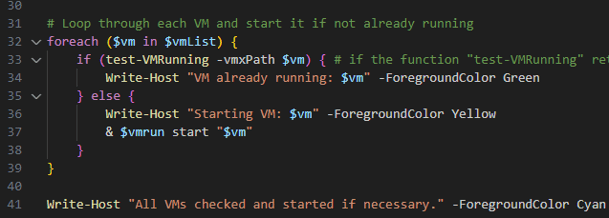
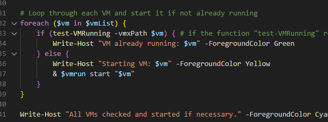
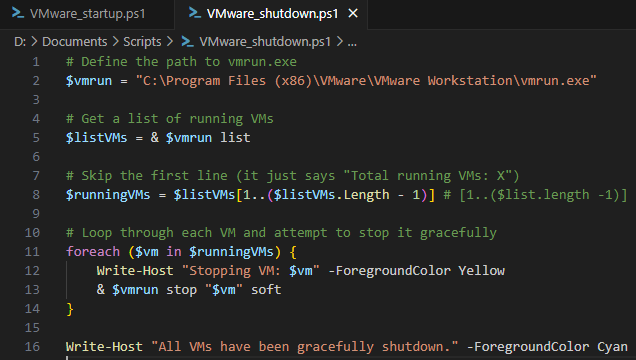
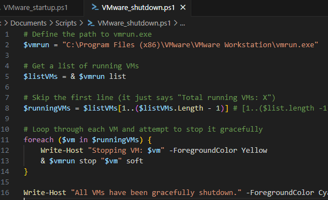
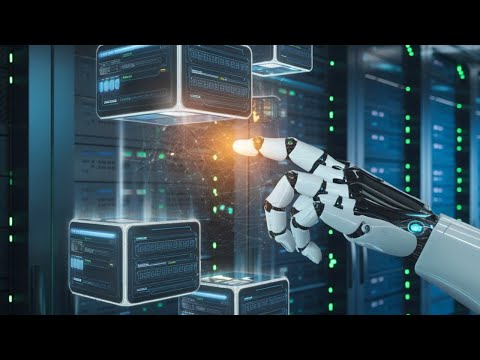